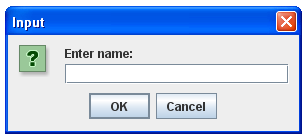
The package that needs to be imported to accept user input is java.io. The java.io package contains classes and interfaces used for input and output.
The setup:
import java.io.*;
class GetUserInput{ }
User input classes
To get user input, use the BufferedReader and InputStreamReader classes.- The InputStreamReader class - reads the user's input.
- The BufferedReader class - buffers the user's input to make it work more efficiently.
- package com.tctalk.myapp.java.src;
- import java.io.BufferedReader;
- import java.io.IOException;
- import java.io.InputStreamReader;
- /**
- * ReaduserInput.java - [This code reads the user input data from command prompt]
- *
- * @author TechCuBeTalk.com
- * @version 1.0
- */
- public class ReaduserInput {
- // Ask the user to enter their name
- // To read user input create a reader object
- String userName = null;
- // read the user input from command prompt by readLine() method
- try {
- userName = br.readLine();
- }
- }
- }
For more practice, here's a demo:
via[techcubetalk.com]
0 comments:
Post a Comment